Willis Tower Constructor Part 2 (Security Management Simulator - Post 3)
Since last time, I added a Service Core constructor to my simple building. The service core is the vertical conduit through which all HVAC, electrical, plumbing, and elevator systems run. My algorithm sets the x and y of the service core independently to be 25-50% the length of the building on the corresponding axis.



I also found out how to change the transparency of the visualizing block so I can see the core in the center. Unfortunately, to really see the core in the middle, the cubes have to be basically invisible, so I tweak them based on what I need to see.
When I left off, I was trying to figure out how use the height values I generated for each square of the tower to actually construct the building. I made an array (mini_building) that is supposed to hold the height value for each square, but I couldn’t figure out how to fill it. I watched a youtube tutorial about procedurally generating a maze multiple times, but once I got my head around it, I realized I didn’t know how to convert into something I could use for my purposes. It uses a recursive backtracker algorithm, which is not what I want. I want it to grow out gradually from a single location, like a blob, not run as far as it can and then come back.
What I wanted vs what I had.
I googled around but with no luck. I’m not experienced enough to figure this out on my own. So I turned to my dreaded enemy. AI.
History may cluck its tongue at me, but hey, I gotta work with what I got! I posed my problem to it and it gave me unhelpful python. But, its methodology did point into the right direction.
Basically I made 3 arrays. One to keep track of where I hadn’t visited, one to keep track of where I had, and a third one to keep track of where it was okay to grow. The algorithm picks a random spot from the visited array (at the beginning there’s only one option: the center). It then checks its neighbors to see if any are unvisited. If they are, it randomly picks one to move into next. Then it moves that one from unvisited to visited and then it repeats until all the tiles are visited. Now “visited“ contains a list of all tile positions, ordered from the ones that should be tallest to the ones that should be shortest.
Next, I needed to use the “visited” array to assign the heights floors. This was again too tricky for me, so I turned back to chatGPT. I had what I needed (1: an array of which tiers have which heights, 2: an array of how many squares are in each tier, and 3: the order that squares get sorted into tiers), I just didn’t know how to pull it together. Thankfully, ChatGPT gave me exactly what I needed. It’s actually really simple.
First, loop through all the coordinates in the “visited” array. At each iteration, use those coordinates select which spot in a 2d array needs to be filled. A counter keeps track of how many squares have been assigned at this level, and a tier tracker tells us which tier we should be on. (They both start at zero.) The counter increments every time a value is assigned, until it equals the number of available squares at that tier. Then we move to the next tier and reset the counter.
After that I could run the building constructor. I just had to interpolate each tile of the large building to where it corresponds to in the smaller building, and use that to determine how tall each column should be. Some code to remap the service core to the center of the tallest tower here, a bit of debugging there, and voilà!
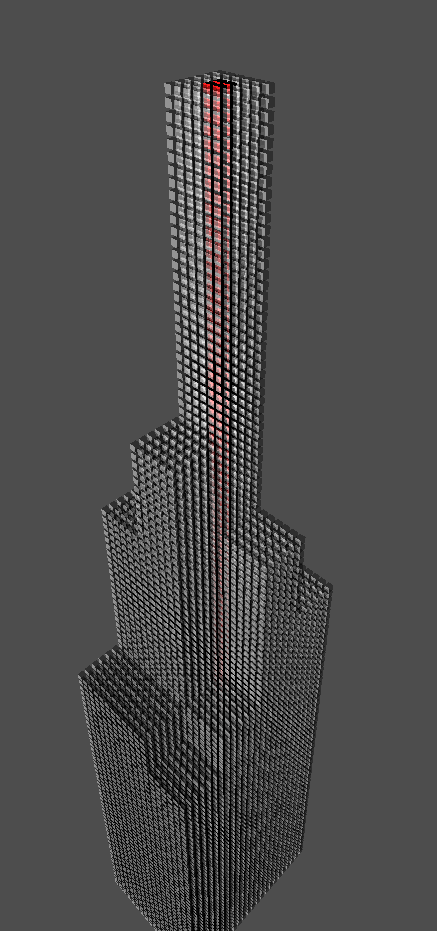

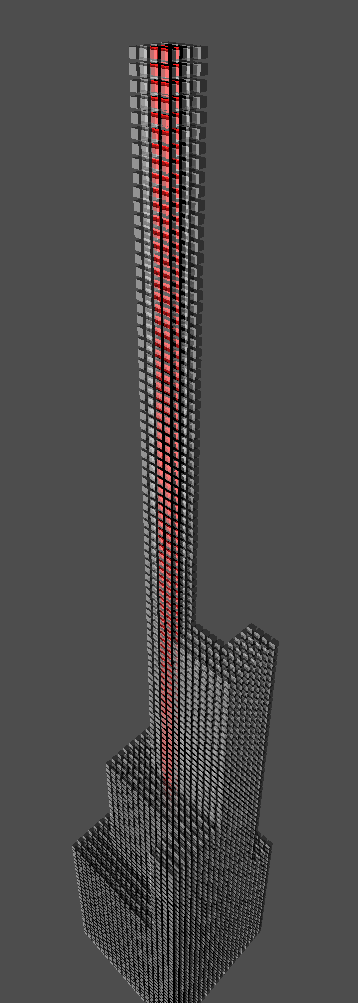
The gap between the tallest and 2nd tallest tier tends to be too large for my liking, so that needs some tweaking. Also the squares should have a higher minimum size; 3x3 is too small.